Introduction to HW Drivers
This topic shows you how to extend the power of FlexOTO with hardware drivers. FlexOTO supports the writing of hardware drivers for optical switches that are not supported by FlexOTO and for measurement instruments such as a power meter. You can install up to eight measurement instruments drivers. These drivers can be written in Python (*.py) or any other language that can compile to an executable file (*.exe), such as C#.
Drivers are easy to write and you'll find all the details and examples within this document. All examples were written and tested in Python. The following figure illustrates the relationship between FlexOTO, a driver, and a non-supported optical switch (or measurement instrument). Additional Python and C# example drivers can be found in the Windows folder Documents\Keysight\FlexOTO\User Drivers.
There is a small possibility that the commands, arguments, and responses described in this document may change. If edits are required for your scripts, they should be minor.
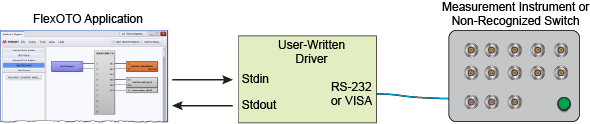
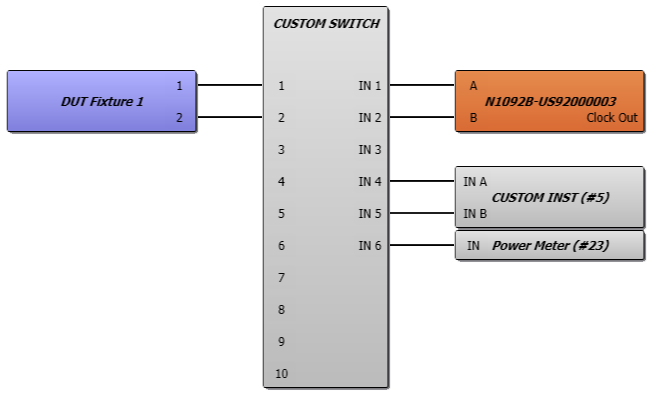
Driver Flowchart
The following flow chart provides the general process for both types of drivers: switch and instrument. Both drivers types have very similar structure. FlexOTO launches your driver from a dialog in which you have entered the driver's file name (along with path) and the COM port or VISA address of the instrument or switch. All communication between FlexOTO and your driver will be passed through stdin and stdout.
The driver runs in a continuous loop waiting for, and reacting to, commands sent from FlexOTO.
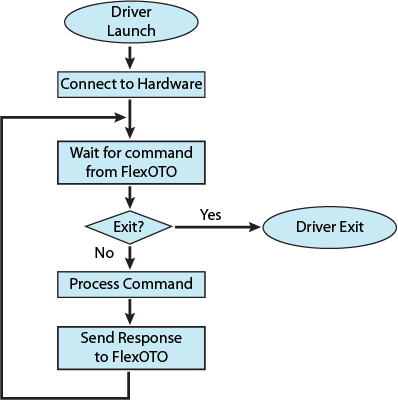
When your driver is launched, FlexOTO immediately sends the get_description
driver command to your driver. This command requests a description of your switch or instrument. This description allows FlexOTO to create a Switch block or Instrument block for the Hardware diagram. Your driver will return the following information in the form of a JavaScript Object Notation (JSON) string:
- Model name of the switch or instrument. In the case of a switch, the names of multiple internal switches can be included.
- Serial number.
- Settling time in seconds (switches only).
- List of switch ports (switches only).
- List of instrument measurement input connectors (instruments only).
In response to a FlexOTO driver command, your driver will either send information back to FlexOTO as a JSON string, translate the command to send to your switch or instrument, or both.
Confirm with the "DONE" string
When your driver completes its initialization or finishes responding to FlexOTO command, which may include returning an error message or data, the driver must afterwards send the "DONE" string. Error messages, data, and the "DONE"
string must be separately sent. The "DONE"
string tells FlexOTO that the response is complete and that the driver will wait for the next command. For example, in response to receiving the get_description
command a switch or instrument driver would send the following:
print(error_message) # if needed print(json_string) # return description of switch or instrument print("DONE")
Error Messages
Errors that occur during initializing or running a driver can result in error messages. Driver initialization includes tasks such as FlexOTO finding and starting your driver, the driver parsing any command line arguments, and the driver establishing a connection to the switch or instrument. Errors are briefly displayed along the bottom of FlexOTO and listed in the Hardware Diagram's Message Log Viewer. To view the log, click Help > View Message Log. Error messages can also be read remotely by sending the :SYSTem:ERRor:NEXT?
SCPI query.
The following are examples of errors that are detected by FlexOTO:
- 122, "File not found."
- 135, "Instrument Error;User driver initialization timed out"
- 136, "User driver command timed out: ""<driver-command>"""
- Example:
"Instrument Error;User driver command timed out: set_routes ""1, 6"""
- 137, "Instrument Error;Unable to parse description JSON: <JSON-error>"
- Example:
"Instrument Error;Unable to parse description JSON: Invalid format"
Errors encountered in the driver itself are reported to FlexOTO by sending the error message via stdout
. If an error occurs, the driver creates the custom string that describes the error, and sends the error message to stdout
. For example, here is the error message created for an incorrect switch or instrument COM port:
rm = visa.ResourceManager() ser = rm.open_resource('COM-FOUR') id = ser.query('*IDN?') if not id: print("Switch COM port is not valid.") # error message print("DONE")
If multiple message print statements were used in the above example, the strings would be concatenated by FlexOTO.
The following errors can be reported from drivers:
- 133, "Instrument Error;Connection failed: Invalid: stdout-string"
- Example:
"Instrument Error;Connection failed Invalid: Only one command line argument allowed."
- 134, 'Instrument Error;User driver command error: "driver-command" returned "stdout-string"'
- Example:
"Instrument Error;User driver command timed out: ""set_routes"" returned ""The switch route could not be made!"""
The maximum length for error messages is 255 characters, but it is recommended to keep your messages short.