Returns and prints results table
This Python example, uses the :MEASurement:RESults?
query to return all of the scalar measurements currently shown in FlexDCA. The query returns a comma-delimited string which the script places into a list of named tuples. Each named tuple represents a measurement row in the table. Because the script locates the name of each field (table column title) it can handle FlexDCA measurement tables that differ in field name and number of fields.
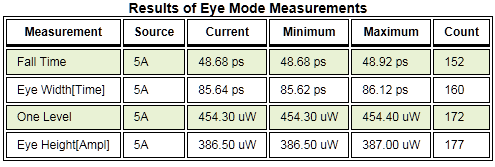
Script
Copy
MEAS-RESults.py
# -*- coding: utf-8 -*-
""" Before running this script, display a waveform on FlexDCA. Start
some measurements so that a measurement results table is displayed.
Depending on the setup, the number of measurement results fields can
vary. This script:
1. Uses :MEAS:RES? to return all measurements shown in table.
3. Creates a list of named tuples, one for each table row.
4. Prints all fields.
"""
import pyvisa as visa # import VISA library
import collections as coll
ADDRESS = 'TCPIP0::localhost::hislip0,4880::INSTR'
Field = coll.namedtuple('Field', 'name value')
def open_flexdca_connection(address):
""" Opens visa connection to FlexFlexDCA. """
print('Connecting to Flexdca ...')
try:
rm = visa.ResourceManager()
connection = rm.open_resource(address)
connection.timeout = 20000 # Set connection timeout to 20s
connection.read_termination = '\n'
connection.write_termination = '\n'
inst_id = connection.query('*IDN?')
print('\nFlexDCA connection established to:\n' + inst_id, flush=True)
except (visa.VisaIOError, visa.InvalidSession):
print('\nVISA ERROR: Cannot open instrument address.\n', flush=True)
return None
except Exception as other:
print('\nVISA ERROR: Cannot connect to instrument:', other, flush=True)
print('\n')
return None
return connection
FlexDCA = open_flexdca_connection(ADDRESS)
results = FlexDCA.query(':MEAS:RESults?').replace(',Name', '\nName')
results_lst = results.split('\n')
FlexDCA.write('SYSTem:GTLocal')
FlexDCA.close()
measurements = []
for measurement in results_lst:
fieldlist = list(measurement.split(','))
list_of_fields = []
for field in fieldlist:
name, value = field.split('=')
list_of_fields.append(Field(name, value))
measurements.append(list_of_fields)
for meas in measurements:
print('*' * 20)
for field in meas:
print('{}: {}'.format(field.name, field.value))