Break Flex-on-Flex Connection from DCA-X
This script demonstrates breaking a Flex-on-Flex connection by sending the :APPLication:RDISconnect
command to the DCA-X. Normally, you would break the connection by sending the :RDCA:DISConnect
command to N1010A FlexDCA on the PC. The following diagram shows a Flex-on-Flex connection. N1010A FlexDCA on a PC connects to a DCA-X.
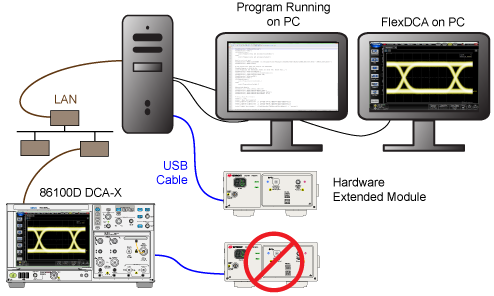
Example Script
Copy
flex-on-flex-break.py
""" This script demonstrates making a Flex-on-Flex connection
(from the PC) and then breaking Flex-on-Flex from the N1000A. The :RDCA subsystem command are used to create
Flex-on-Flex, and the :APPLication subsystem commands are used to
disconnect Flex-on-Flex.
"""
import pyvisa as visa # import VISA library
FLEX_ADDRESS = 'TCPIP0::localhost::hislip0,4880::INSTR'
DCAX_ADDRESS = 'TCPIP0::K-N1000A-00240::hislip0,4880::INSTR'
DCAX_HOSTNAME = 'K-N1000A-00240'
def open_flexdca_connection(address):
""" Opens visa connection to FlexFlexDCA. """
print('Connecting to Flexdca ...')
try:
rm = visa.ResourceManager()
connection = rm.open_resource(address)
connection.timeout = 20000 # Set connection timeout to 20s
connection.read_termination = '\n'
connection.write_termination = '\n'
inst_id = connection.query('*IDN?')
print('\nFlexDCA connection established to:\n' + inst_id, flush=True)
except (visa.VisaIOError, visa.InvalidSession):
print('\nVISA ERROR: Cannot open instrument address.\n', flush=True)
return None
except Exception as other:
print('\nVISA ERROR: Cannot connect to instrument:', other, flush=True)
print('\n')
return None
return connection
def connect_FlexDCA_to_DCAX(FlexDCA, DCAX_HOSTNAME):
"""Opens connections from FlexDCA on PC to N1000A's FlexDCA. """
print('Connecting to DCA-X. Please wait...', flush=True)
FlexDCA.write(':RDCA:CONNect:METhod LAN')
FlexDCA.write(':RDCA:CONNect:MODE STANdard')
FlexDCA.write(':RDCA:CONNect:ACTion TBSettings')
# Pull state upon connect
FlexDCA.write(':RDCA:CONNect:TSETtings ON')
# Push state upon disconnect
FlexDCA.write(':RDCA:DISConnect:TSETtings ON')
FlexDCA.write(':RDCA:CONNect:HOST "' + DCAX_HOSTNAME + '"')
if FlexDCA.query(':RDCA:CONNect;*OPC?'):
print(FlexDCA.query('*IDN?'))
def view_message():
""" """
print('-' * 20)
s = 'Confirm that FlexDCA on the PC is now connected to the \
N1000A. FlexDCA on your PC should now be \
showing the DCA-X\'s screen.\n\nNote: In this mode, if you install \
a simulated module, it will be installed on FlexDCA on the PC not \
on the DCA-X.\
\n\nTo send a command to the DCA-X to disconnect from FlexDCA \
on the PC, press the Enter key:'
input(s)
def disconnect_flex_on_flex(DCAX):
s = DCAX.query('*IDN?')
print('\nBreaking Flex-on-Flex from DCA-X:\n\t' + s, flush=True)
if DCAX.query(':APPLication:CSTate?') in '1':
s = DCAX.query(':APPLication:CNAMe?')
print('\tConnection made by: ', s)
DCAX.write(':APPLication:RDISconnect')
print('\nFlex-on-Flex disconnected from DCA-X.')
else:
print('\nA Flex-on-Flex connection does not exist to this DCA-X.')
print('Ending program.')
FlexDCA = open_flexdca_connection(FLEX_ADDRESS)
connect_FlexDCA_to_DCAX(FlexDCA, DCAX_HOSTNAME)
FlexDCA.write(':SYSTem:GTLocal')
view_message()
DCAX = open_flexdca_connection(DCAX_ADDRESS)
disconnect_flex_on_flex(DCAX)
DCAX.write(':SYSTem:GTLocal')
DCAX.close()
FlexDCA.write(':SYSTem:GTLocal')
FlexDCA.close()