Example 2. Show Input Variables
This example and all remaining examples, include a setup Python script that configures FlexDCA so that the example user measurement script has all the conditions needed to successfully run.
This example introduces how to print to FlexDCA's User Measurement Output dialog. To view this dialog,
- Click the User Meas button on FlexDCA's toolbar to show the User Measurement Setup dialog.
- In dialog, click the Show Output button which opens the User Measurement Output dialog.
After running the script, if the Show Output button is not shown on the User Measurement Output dialog or no text has been written to the User Measurement Output dialog:
- Close and then reopen the dialogs to update them.
- Click FlexDCA's Single button to run a data acquisition. Each time that you click Single the script runs again and prints to the Show Output dialog. If there are no print statements in your script, Show Output will not be displayed.
You may never use this feature, but it is valuable to have in your toolbox. The script prints to the User Measurement Output dialog the top-level contents of the input variables (list of dictionaries) that are passed the your script's algorithm function. This script does not perform a user measurement but does return a fictitious measurement (4.2) as required by FlexDCA.
The Show Output dialog cannot display Unicode symbols such as Δ. After you've been successful with this example, try the Example 3. Save Dependent Measurement Data.
Because Example 1 showed how to install a user measurement, this and the remaining examples will not repeat this procedure.
Before running this example script, run the ShowInputVars-setup.py
script which configures FlexDCA with the measurement mode, waveform, and dependent measurements that are required by Example 2.
Example of User Measurement Output dialog
This dialog is updated with each data acquisition. Click FlexDCA's Single button to stop the updates.
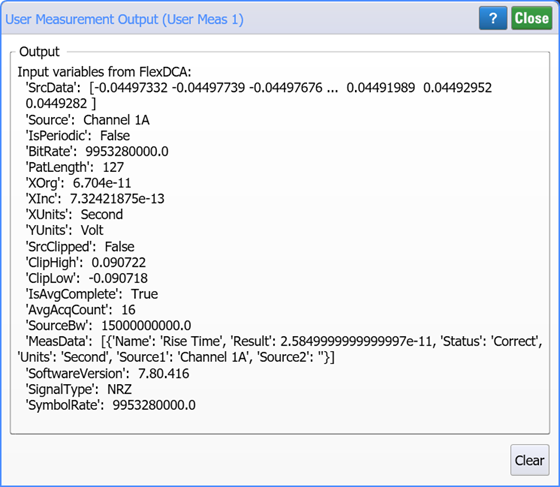
Required Files
ShowInputVars-setup.py
This script configures FlexDCA to required state for the example script. The script installs a simulated module, selects the proper FlexDCA mode, and turns on a rise-time measurement.
ShowInputVars-setup.py
import pyvisa as visa # import VISA library
visa_address = 'TCPIP0::localhost::hislip0,4880::INSTR'
CHANNEL = '1A'
TIMEOUT = 10000
def open_flexdca_connection(address):
""" Opens visa connection to FlexFlexDCA. """
print('Connecting to Flexdca ...')
try:
rm = visa.ResourceManager()
connection = rm.open_resource(address)
connection.timeout = TIMEOUT # Set connection timeout to 20s
connection.read_termination = '\n'
connection.write_termination = '\n'
inst_id = connection.query('*IDN?')
print('\nFlexDCA connection established to:\n' + inst_id, flush=True)
connection.write(':SYSTem:DEFault')
connection.query('*OPC?')
except (visa.VisaIOError, visa.InvalidSession):
print('\nVISA ERROR: Cannot open instrument address.\n', flush=True)
return None
except Exception as other:
print('\nVISA ERROR: Cannot connect to instrument:', other, flush=True)
print('\n')
return None
return connection
def all_channels_off(FlexDCA):
""" Turns all available channels off. """
for slot in '12345678':
for letter in 'ABCD':
channel = slot + letter
FlexDCA.write(':CHANnel' + channel + ':DISPlay OFF')
def install_simulated_module(FlexDCA, channel, model, signal='NRZ'):
""" Simplified installation of a simulated FlexDCA module.
model
Type of simulated module. "DEM" is a dual-electrical module.
"QEM" is a quad-electrical module. "DOM" is a dual-optical
module. "QEM" is a electrical-optical module.
signal
Format of signal. NRZ or PAM4.
"""
slot = channel[0]
FlexDCA.write(':EMODules:SLOT' + slot + ':SELection ' + model)
if signal in 'NRZ':
FlexDCA.write(':SOURce' + channel + ':FORMat NRZ')
else:
FlexDCA.write(':SOURce' + channel + ':FORMat PAM4')
FlexDCA.write(':SOURce' + channel + ':DRATe 9.95328E+9')
FlexDCA.write(':SOURce' + channel + ':WTYPe DATA')
FlexDCA.write(':SOURce' + channel + ':PLENgth 127')
FlexDCA.write(':SOURce' + channel + ':AMPLitude 90E-3')
FlexDCA.write(':SOURce' + channel + ':NOISe:RN 3.0E-6')
FlexDCA.write(':SOURce' + channel + ':JITTer:RJ 4.0E-12')
FlexDCA.write(':CHANnel' + channel + ':DISPlay ON')
def return_scalar(FlexDCA, query):
""" Returns a FlexDCA scalar measurement result. """
import time
class ScalarMeasQuestionableException(Exception):
""" A scalar measurement result is questionable. """
pass
class ScalarMeasTimeOutException(Exception):
""" A scalar measurement has timed out. """
pass
timeout = FlexDCA.timeout / 1000 # convert ms to s
query = query.strip('?')
start_time = time.time() # time in seconds
while(True):
time.sleep(0.2)
status = FlexDCA.query(query + ':STATus?')
if 'CORR' in status: # valid measurement result
return FlexDCA.query(query + '?') # get measurement
elif 'QUES' in status: # questionable results
s = query + '\nReason: ' + FlexDCA.query(query + ':STATus:REASon?')
raise ScalarMeasQuestionableException(s)
elif (int(time.time() - start_time)) > timeout: # IO timout
s = query + '\nReason: ' + FlexDCA.query(query + ':STATus:REASon?')
raise ScalarMeasTimeOutException(s)
flexdca = open_flexdca_connection(visa_address)
flexdca.query(':SYSTem:DEFault;*OPC?')
all_channels_off(flexdca)
install_simulated_module(flexdca, CHANNEL, 'DEM')
flexdca.write(':ACQuire:RUN')
flexdca.query(':SYSTem:MODE OSCilloscope;*OPC?') # Switch to Eye/Mask mode
flexdca.query(':SYSTEM:AUToscale;*OPC?')
flexdca.query(':TRIGger:PLOCk ON;*OPC?')
flexdca.write(':ACQuire:SMOothing AVERage')
flexdca.write(':TIMebase:SCALe 1.500E-10')
flexdca.write(':MEASure:OSCilloscope:RISetime')
flexdca.write(':SYSTem:GTLocal')
flexdca.close()
print('On FlexDCA in scope mode:')
print('\t1. Click on User tab and click on User Meas Setup button.')
print('\t2. Browse to and load ShowInput Vars.xml into User Measurement 1 tab.')
print('\t3. Close the User Measurement Setup dialog.')
print('\t4. On the User tab, click User Meas 1.')
print('\t5. User Measurement Setup dialog and click Show Output.')
print("\t6. Near FlexDCA's menu bar, Click Single to perform a single waveform acquisition.")
print('\t7. The input variables passed into ShowInputVars.py will be listed.')
ShowInputVars.xml
Installs user measurement.
ShowInputVars.xml
<?xml version="1.0" encoding="utf-8"?>
<Measurement>
<Name>Show Input Vars</Name>
<Abbreviation>InVars</Abbreviation>
<Comments>Writes input variables and their values to the Show Output window.
</Comments>
<Script>ShowInputVars.py</Script>
<MeasurementType>1 Source</MeasurementType>
<ValidMode>Scope</ValidMode>
<Dependent Name='Rise Time'></Dependent>
</Measurement>
ShowInputVars.py
User measurement script. Notice that this script includes a handy print_to_show_window()
function that increases the reliability of writing to the Show Output window. This function replaces Unicode characters, which cause an error, with the equivalent XML character entity. For example, the Δ symbol in the name of the "Δ Time" measurement is replaced with the entity string.
ShowInputVars.py
"""
Python 3.9 FlexDCA User Measurement
Print the keys and values of the dictionary, that is passed from FlexDCA to this,
script, to the Show Output window. Since Show Output does not accept any
Unicode characters, the print_to_show_window() function removes them.
The Show Output window also truncates lines over 80 characters, but this
function does not correct for this.
"""
def algorithm(inputVars):
print('Input variables from FlexDCA:')
for var, value in inputVars.items():
s = " '{0}': {1}".format(var, value)
print_to_show_window(s)
return {'Result': 4.2,
'Units':'dB',
'Status':'Correct',
'ErrorMsg':''}
def print_to_show_window(message):
""" FlexDCA's Show Output window for user measurements and operators
causes a error if a Unicode character is in the printed string.
Removes all Unicode characters. It also removes any '\n' and '\t'
characters.
"""
message = message.replace('\n', '1banana')
message = message.replace('\t', '2squirrels')
asciiString = message.encode('ascii', 'xmlcharrefreplace')
cleanMessage = asciiString.decode('utf-8')
cleanMessage = cleanMessage.replace('1banana', '\n')
cleanMessage = cleanMessage.replace('2squirrels', '\t')
print(cleanMessage)